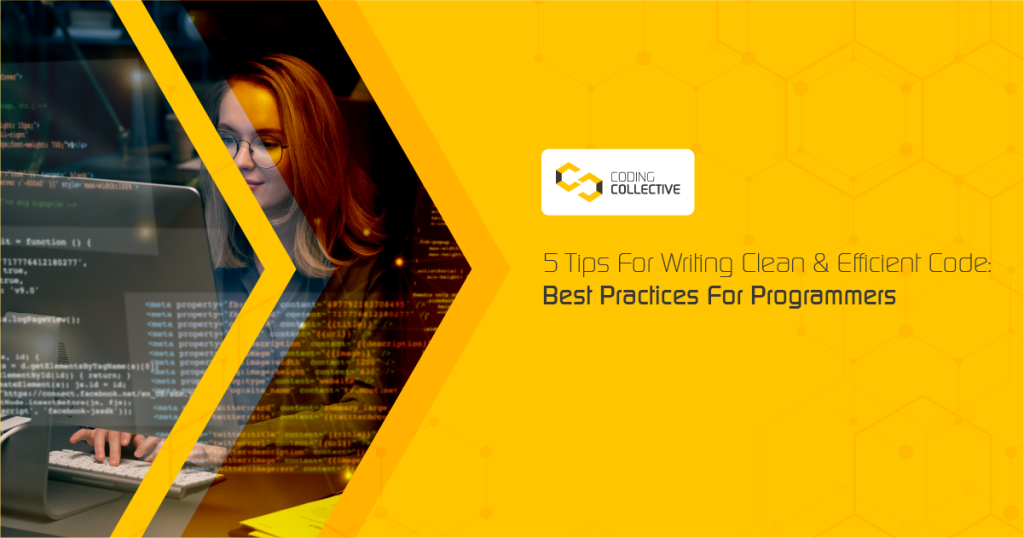
Introduction
Writing clean and efficient code is not just a best practice for programmers, it is a fundamental skill that separates good programmers from the great ones. Clean code is easy to read and understand, while efficient code is optimized for performance and scalability. In this blog, we will discuss five tips for writing clean and efficient code that will help you become a better programmer.
1. Use Descriptive and Meaningful Names
One of the most important aspects of writing clean code is using descriptive and meaningful names for your variables, functions, and classes. The names you choose should reflect the purpose and functionality of the code they represent. For example, instead of using names like “x,” “y,” and “z” for variables, use descriptive names like “totalSales,” “average rating,” and “numItemsSold.” This makes it easier for other developers to understand your code, and it also makes it easier for you to remember what your code does if you come back to it later.
Another aspect of naming conventions is to use consistent naming throughout your code. For instance, if you use camelCase for variable names, stick to that convention and don’t mix it with snake_case or PascalCase. Consistent naming conventions make your code more readable and maintainable, which is essential for writing clean code.
2. Follow the DRY Principle
The DRY principle stands for “Don’t Repeat Yourself,” which means that you should avoid duplicating code as much as possible. If you find yourself writing the same code in multiple places, it is a sign that you need to refactor your code and create reusable functions or classes. This not only makes your code more efficient, but it also makes it easier to maintain and modify in the future.
For example, let’s say you have a web application that calculates the average rating of a product based on user reviews. Instead of writing the code to calculate the average rating in multiple places throughout your application, create a function that can be called from anywhere in your code. This makes your code more efficient and reduces the chances of introducing errors if you need to make changes to the code in the future.
3. Keep Your Functions and Classes Small and Focused
Another best practice for writing clean and efficient code is to keep your functions and classes small and focused. This means that each function or class should have a single responsibility and do one thing well. When functions or classes become too large and complex, it becomes difficult to understand and maintain the code.
One way to keep your functions and classes small is to use the Single Responsibility Principle (SRP). The SRP states that each function or class should have only one reason to change. This means that if you need to make changes to a function or class, it should be for one specific reason, such as adding a new feature or fixing a bug. By following the SRP, you can create more modular and reusable code that is easier to maintain.
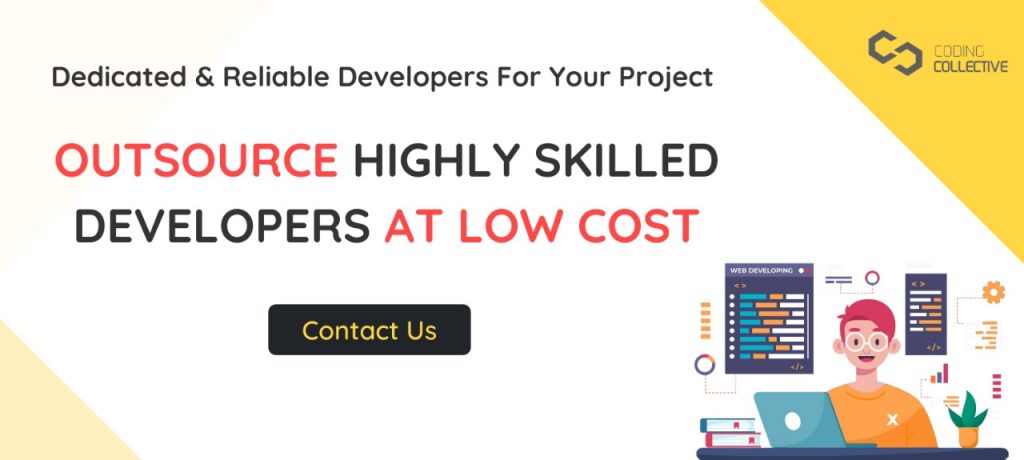
4. Write Readable Code
Readable code is essential for writing clean and efficient code. Readable code is code that is easy to understand, even for someone who is not familiar with the codebase. It is important to use consistent indentation, whitespace, and formatting to make your code more readable. Additionally, it is a good practice to use comments to explain complex logic or to provide context for your code.
Another best practice for writing readable code is to use meaningful variable names and avoid abbreviations or acronyms that are not commonly used. It is also a good idea to break down complex logic into smaller, more manageable pieces that are easier to understand.
5. Optimize Your Code for Performance
One way to optimize your code is to use data structures that are optimized for the specific task you are performing. For example, if you are working with large amounts of data, you may want to use a data structure like a hash table or a tree that can handle large amounts of data efficiently. If you are working with small amounts of data, you may want to use a simpler data structure like an array or a linked list.
Another way to optimize your code is to avoid unnecessary computations. For example, if you are iterating through a list of numbers and you only need to perform a computation on a subset of those numbers, you can use a conditional statement to skip over the numbers that you don’t need to compute. This can save a significant amount of processing time and make your code more efficient.
Finally, reducing memory usage is another important way to optimize your code for performance. One way to do this is to avoid creating unnecessary variables or data structures that take up memory. Additionally, you can use techniques like lazy loading or caching to avoid reloading data that has already been loaded into memory.
Conclusion
Writing clean and efficient code is an essential skill for any programmer. By following the tips outlined in this blog, you can create code that is easy to read, maintain, and optimize for performance. Remember to use descriptive and meaningful names, follow the DRY principle, keep your functions and classes small and focused, write readable code, and optimize your code for performance. With these best practices in mind, you can become a better programmer and create code that is efficient, effective, and easy to maintain.
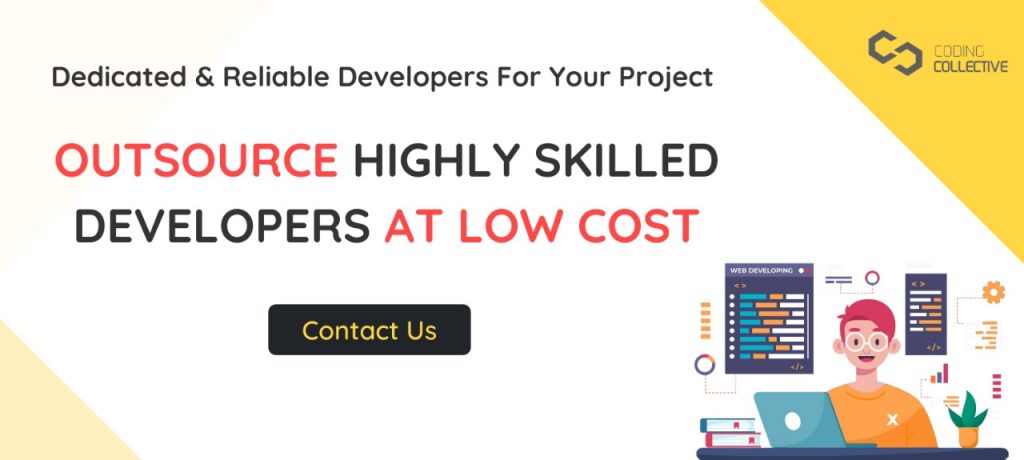